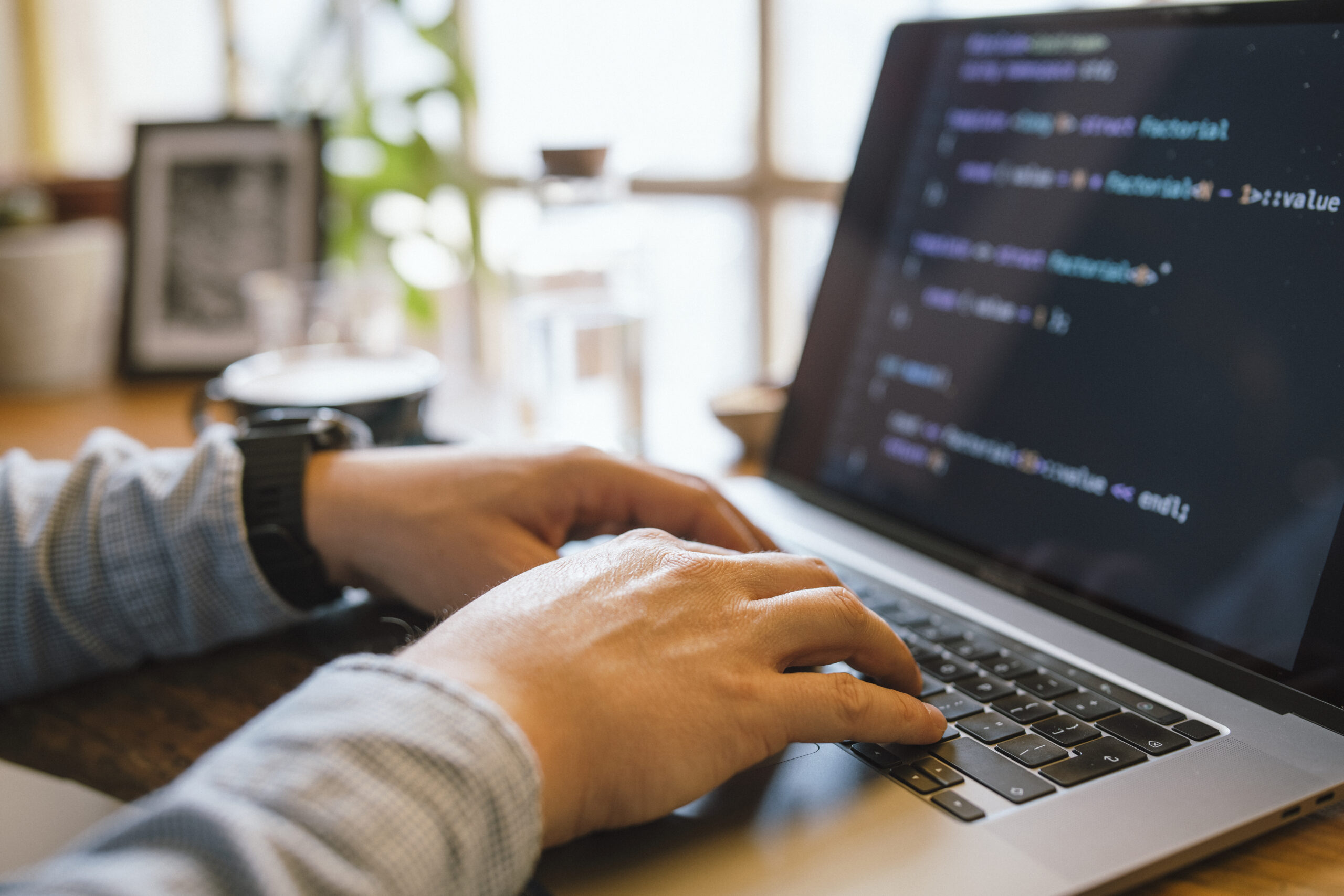
Debugging is One of the more important — still normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of advancement, understanding how to connect with it efficiently throughout execution is Similarly critical. Contemporary development environments appear equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these applications can do.
Take, such as, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code about the fly. When utilized effectively, they let you notice exactly how your code behaves through execution, that is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, observe network requests, watch real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into workable duties.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these tools could have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle programs like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not dropped in the dead of night. The better you understand your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
The most essential — and sometimes ignored — steps in effective debugging is reproducing the condition. Right before leaping in to the code or creating guesses, builders will need to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in squandered time and fragile code adjustments.
The initial step in reproducing a problem is collecting just as much context as you possibly can. Check with inquiries like: What actions brought about The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The greater detail you have, the much easier it turns into to isolate the precise problems below which the bug takes place.
After you’ve gathered sufficient facts, try and recreate the problem in your neighborhood atmosphere. This might imply inputting the exact same information, simulating very similar user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These checks not only support expose the problem but in addition reduce regressions Later on.
Often, The difficulty might be setting-unique — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. That has a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes securely, and communicate much more clearly together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s wherever developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should learn to take care of error messages as direct communications from the procedure. They generally let you know just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Lots of developers, especially when underneath time strain, look at the primary line and right away start building assumptions. But deeper during the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of improvement, INFO for general situations (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure significant messages and slow down your method. Deal with critical activities, state improvements, input/output values, and important final decision points in the code.
Format your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical activity—it is a form of investigation. To efficiently detect and fix bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking allows break down complicated concerns into workable pieces and follow clues logically to uncover the root result in.
Commence by collecting proof. Consider the signs or symptoms of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire just as much appropriate data as you may without the need of leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Up coming, type hypotheses. Talk to yourself: What could be producing this actions? Have any improvements not long ago been manufactured on the codebase? Has this concern occurred before less than very similar conditions? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Try to recreate the condition in a very controlled environment. For those who suspect a certain perform or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out near attention to smaller particulars. Bugs normally cover in the minimum expected destinations—like a lacking semicolon, an off-by-1 mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and aid Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Exams
Composing assessments is among the most effective methods to increase your debugging techniques and overall improvement efficiency. Exams not simply enable capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-tested software is much easier to debug because it allows you to pinpoint precisely in which and when a difficulty happens.
Begin with unit exams, which target specific features or modules. These tiny, isolated exams can swiftly reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glimpse, appreciably cutting down enough time invested debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These assist ensure that many portions of your application work jointly easily. They’re particularly handy for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Writing assessments also forces you to definitely Consider critically about your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the take a look at fails continuously, you'll be able to deal with fixing the bug and look at your test pass when The problem is fixed. This strategy makes sure that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a discouraging guessing activity into a structured and predictable method—encouraging you catch much more bugs, more rapidly plus more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to become immersed in the trouble—observing your monitor for several hours, attempting Resolution immediately after Alternative. But Probably the most underrated debugging equipment is actually stepping absent. Getting breaks can help you reset your head, cut down frustration, and often see the issue from a new perspective.
When you're as well close to Gustavo Woltmann coding the code for as well extended, cognitive fatigue sets in. You could start off overlooking clear glitches or misreading code that you just wrote just hrs previously. On this state, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a smart method. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can educate you anything precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What prompted it? Why did it go unnoticed? Could it are actually caught earlier with better practices like unit testing, code reviews, or logging? The answers frequently reveal blind spots in your workflow or understanding and assist you to Develop stronger coding routines moving forward.
Documenting bugs will also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the best developers are usually not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.